I just finished up my design of EKG gizmo. Thus far, it’s setup for only One IR sensor (but can be expanded).
I learned a lot about the “Finickyness” IR from this project and provide some comments that may help:
• Using Elegoo Nano (and Elegoo UNO’s). Both work. For the Nano, it’s important to select the “Old Bootloader”
• I don’t use (or like) most libraries provided by users in Arduino - they are often specific to board&chip and IDE versions when the Open-Source author uploaded it. (I prefer doing my own code - most often in C++.)
• IR emitters (IR_LED’s, etc) are most often Paired with IR receivers - meaning, mixing Brands of IR sensors isn’t always successful.
• “Important” Most ‘User’ schematics show IR_Receiver Incorrectly! Check yours.
• There are two “AND” syntax’s: One for Logical operations and One for Bitwise operations. They are NOT mixable with certainty of outcome. Use the correct one.
“&” is for Bitwise
“&&” is for Logical
Also, Arduino IDE syntax changes in released versions so, I suggest placing a ‘space’ between ‘&’ or ‘&&’ and ‘Results. i.e., “ && results, Not “&&results”
I recommend starting with a simple AnalogRead of your IR_Receiver. The IR_Emitter can be supplied with a constant ‘High’ to keep it on - Be Sure to Use a Resistor - check spec’s for correct value. Easy to blow out an IR.
Most cameras (including Cellphones) will see the IR_emitter light as purple. That way, you’ll know if emitting. Receiver may also show purple.
IR hook-up and loading can be fussy and easy to blow them without proper resistance. Sure, you can get them to work then, after a few minutes, you’ll smell burning…
Note: I’m using an LM358 OpAmp for this project.
Images:
IR Emit & Rcvr on BB with LM358 to condition signal
IR On showing Purple
Gizmo on BB (Yes, my pulse is 50! That’s what got me started on an EKG project:laughing:
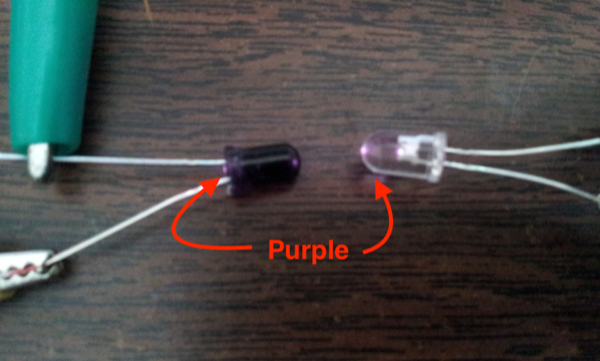